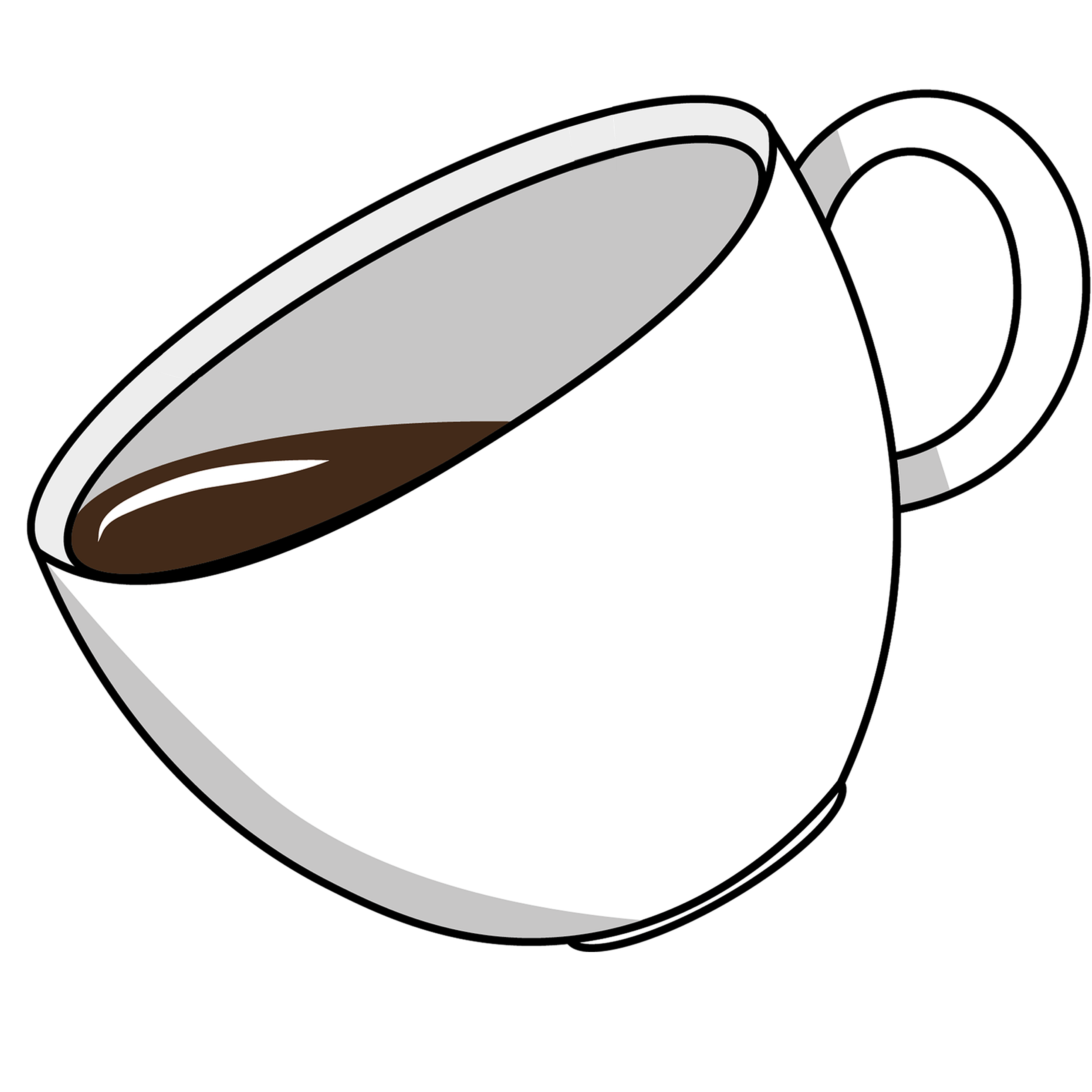
In our previous how-to, we walked through how to setup your Rest API with Node.js and Express.js. Don't worry if you missed it! The project setup should only take a couple minutes and you can find it here. In todays how-to we will walk through how to create a connection to MongoDB — and perform CRUD (Create, Request, Update, and Delete) functions for a simple online store application. Now this how-to will require you to have Mongo installed on your machine, and so were not working in a terminal we will be installing a GUI for MongoDB being MongoDB Compass.
One of the many perks of implementing MongoDB as your database store with Node.js, is being able to utilize the Mongoose library. Mongoose is a Object Data Modeling library that we can install into our project to streamline the data management process between our Node.js REST API, and our MongoDB collections. Sounds pretty cool right? Let's get started!
We will need to install the Mongoose library into our existing project, so navigate into your root directory and execute the following command:
npm i mongoose
If Mongoose successfully installed you'll be able to see it in your "package.json" file as shown below.
{ "name": "myrestapi", "version": "1.0.0", "description": "my node api", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1", "start": "node app.js" }, "author": "corey domingos", "license": "ISC", "dependencies": { "express": "^4.17.2", "mongoose": "^6.1.8" } }
With Mongoose successfully installed, follow this guide to setup your local MongoDB instance and create a database using Compass if you wish to do so. Now with the local MongoDB instance setup, lets structure our project to handle the incoming requests we will be building out in this how-to. At your project root directory, create three directories being "controllers", "models", and "routes". In the "models" directory create a JavaScript file labeled "db.js". In the "db.js" file we will create our connection to our MongoDB instance to look like below:
Note: If you are building a production level application, your MongoDB URI should be stored in some sort of secure file storage live a ".env" or "config" file to avoid security breaches.
//require mongoose var mongoose = require('mongoose'); //our mongo connection URI var mongoDB_URI = 'mongodb://localhost:27017/RestApi'; //create our connection to the local MongoDB instance mongoose.connect(mongoDB_URI, (err) => { if (!err) { //alert if a successful connection is made console.log('Successful MongoDB connection') } else { //alert if there is an error in the connection console.log("Error connecting to MongoDB: " + JSON.stringify(err, undefined, 2)); } } );
Modify the existing "app.js" file to require our new "db.js" file as below:
var express = require('express'); var app = express(); var port = 8080; //added to require the MongoDB config require('./models/db'); app.get('/testApi', (req, res) => { res.status(200).json({status: true, messsage : "The api is working!"}) }) app.listen(port, () => { console.log(`Server is listening on port: ${port}`) })
Execute the start command for the app in your terminal:
node app.js
If all is well you should be getting two logs in your console, one for our existing server notification to tell us that the server is live, and one for our newly created MongoDB connection to our local instance.
Server is listening on port: 8080 Successful MongoDB connection
Now that we have a successful connection to our local MongoDB instance — lets define a mongoose model. In the "models" directory, create a new file named "basic.js" and modify like below:
//require mongoose const mongoose = require('mongoose'); //define our collection to write to our data base var basicSchema = new mongoose.Schema({ title: String, price: Number, quantity: Number, productId: Number }) //export the model mongoose.model('Basic', basicSchema);
With the model defined, we need to give the "db.js" file access to this file by adding the below to the "db.js" file:
require('./basic');
With the Basic model defined it's time to configure some Create, Request, Update, and Delete endpoints for the REST API to handle. In the "routes" directory create a file "basic.router.js" to handle our incoming Client Side requests (in this case it would be the REST client), and in the controllers directory create a file named "basic.controller.js" to handle the associated logic for each API endpoint. Now to read the incoming JSON request from the Client side we will need to install body-parser with the below command:
npm i body-parser
With body-parser installed, let's import mongoose into the controller and require the Basic model that we defined above.
//require mongoose const mongoose = require('mongoose'); //require the basic model const Basic = mongoose.model('Basic');
Now in the same "basic.controllers.js" file, we'll add in the methods to handle the logic for creating a new item, requesting the items, updating an item, and deleting an item like below:
//Method to handle creating a new record module.exports.createItem = (req, res) => { //create an instance of our model var basic = new Basic() //assign the fields defined in the model to the incomming request data basic.title = req.body.title; basic.price = req.body.price; basic.quantity = req.body.quantity; basic.productId = req.body.productId; //save the new document basic.save((err, obj) => { if (err) { //return err response if incountered res.status(422).json({status: false, error: "Error in creating item"}); } else { //return success response res.status(200).json({status: true, message: "Item successfully created"}); } }) } //method to handle getting all items module.exports.getItems = (req, res) => { //get all items in the collection Basic.find() .then((items) => { if (!items) { //no documents found return error res.status(404).json({status: false, error: "No items found"}); } else { //return the items in the response res.status(200).json({ items }); } }) } //method to handle updating the quantity of a item module.exports.updateItem = (req, res) => { //find the item to update Basic.findOneAndUpdate({productId : req.params.id}, {$set : { quantity: req.body.quantity}}, {new : true}, (err, updatedObj) => { if (err) { res.status(422).json({status : false, error : "Item not updated"}); } else { res.status(200).json({ updatedObj }); } }) } //method to handle deleting an item module.exports.deleteItem = (req, res) => { //find the item we want to delete Basic.findOneAndDelete({productId : req.params.id}, (err, deletedObj) => { if (err) { res.status(404).json({status: false, error: "Item not found"}); } else { res.status(200).json({status: true, message: "Item successfully deleted"}); } }) }
Now we can update the "basic.router.js" file in the "routes" directory to implement these methods:
//require express const express = require('express'); //instance of express router const router = express.Router(); //instance of the basic controller const Basic = require('../controllers/basic.controller'); //define the CRUD routes router.post('/createItem', Basic.createItem); router.get('/getItems', Basic.getItems); router.put('/updateItem/:id', Basic.updateItem); router.delete('/deleteItem/:id', Basic.deleteItem); //export router module.exports = router;
With this done, we just have to make some alterations to our "app.js" to make use of the new router files and the body-parser package we installed. The "app.js" should be modified like below:
var express = require('express'); var app = express(); var port = 8080; const bodyParser = require('body-parser'); require('./models/db'); const basicRouter = require('./routes/basic.router'); //instance of the express app & implement BodyParser var app = express(); app.use(bodyParser.json()); //allow access to the router file app.use('', basicRouter); app.get('/testApi', (req, res) => { res.status(200).json({status: true, messsage : "The api is working!"}) }) app.listen(port, () => { console.log(`Server is listening on port: ${port}`) })
Recompile the server with the start command:
node app.js
With the Mongo Schema, route handling, and method logic built to handle our four REST API endpoints at:
We can now test these endpoints with our REST Client tool to insure that they are working properly.
Create request
In your REST Client, make a POST request to "localhost:8080/createItem". Now the fields that the createItem() method in the 'basic.controller.js" file is going to be expecting to come from this request are "title", "price", "quantity", and "productId" — so we need to make sure the REST Client is sending these fields in the body. Input the below into the body section of the POST request being sent by the REST Client:
{ "title" : "shirt", "price" : "100", "quantity" : "10", "productId" : "1" }
After this POST request is made, you should be able to view this document created in the Basic Schema in MongoDB Compass — or terminal depending on your use case. Additionally we can check to see if the request was successful by checking the response in the REST Client. If successful you should see the below:
{ "status": true, "message": "Item successfully created" }
Get request
In your Rest Client, make a GET request to "localhost:8080/getItems". You should see the below in the REST Client response:
{ "items": [ { "_id": "61f35a22acfe3911ff83e5ed", "title": "shirt", "price": 100, "quantity": 10, "productId": 1, "__v": 0 } ], }
Update request
In your Rest Client, make a PUT request to "localhost:8080/updateItem/1", where "1" is the "productId" for the record that we are looking to update. Now the method updateItem() in the "basic.controller.js" file is going to be looking for us to pass it a new "quantity" value, so structure your PUT request body in your REST Client like below to update the quantity:
{ "quantity" : "9" }
After this PUT request is made, the returned document should reflect "9" for the quantity as below:
{ "updatedObj": { "_id": "61f35a22acfe3911ff83e5ed", "title": "shirt", "price": 100, "quantity": 9, "productId": 1, "__v": 0 } }
Delete request
In your REST Client make a DELETE request to "localhost:8080/deleteItem/1", where "1" is the "productId" for the record that we are looking to delete. You should see the below in the REST Client response:
{ "status": true, "message": "Item successfully deleted" }
In just a couple easy steps we have updated our existing REST API to handle Create, Request, Update, and Delete endpoint calls to perform REST API functions on documents in MongoDB. Pretty cool right? The source code for this project can be found on my Github here.
Interested in creating a conversation on this blog post? Create a post in our Node.js community!
While client side development can be fun and lucrative, expanding your abilities into API development will turn you into a more well rounded Software Developer. APIs, or Application Programming Interfaces, allow software systems to transmit data between one an other, and promote highly reusable systems. Since the inception of REST architecture in 2000 by Roy Fielding, a new standard was set for API development — which began to really gain traction in 2005 and is still an extremely relevant architecture to this day. A variety of languages can be used to create REST APIs, but today we will walk through how to create a REST API with Node.js and Express.
API development is by far my favorite aspect of Software Development, and if you share the same lust to understand what is going on behind the scenes — I'm sure you'll grow to share this same sentiment towards API development. REST, less commonly known as, Representational State Transfer is a API development Architecture that is used to handle HTTP requests to typically transfer, modify, or manipulate resources. With REST architecture you'll be able to make use of the following HTTP requests to perform these actions:
- GET (request from a resource)
- POST (publish to a resource)
- PUT (publish or update a resource)
- Patch (modify a resource)
- Delete (remove the resource)
These actions will be tied to what we refer to as a API endpoint — which is the resource that you are trying to access in the form of an address. Each unique API endpoint will implement your desired API level logic on the requested resource. Enough background, lets setup a REST API using Node.js that we will be able to build on in future how-to's.
First things first we will need Node.js and NPM installed on your machine, if you do not you can download them here. To check if you have Node.js and NPM already installed, run the below commands in your command prompt to verify.
node -v npm -v
In your command prompt navigate to the sub directory where you would like your API to be stored and run:
mkdir myRestApi
With our project folder created we can now navigate into our project and run:
npm init
This will then walk us through creating the "package.json" file.
Press ^C at any time to quit. package name: (myrestapi) version: (1.0.0) description: my node api entry point: (app.js) test command: git repository: keywords: author: your name license: (ISC) About to write to C:\Users\yourdir\package.json: { "name": "myrestapi", "version": "1.0.0", "description": "my node api", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "author": "corey domingos", "license": "ISC" } Is this OK? (yes)
After the "package.json" file is created we will install Express using:
npm i express
Now we can open our project in Visual Studio Code by executing:
code .
With the project open in VS Code, open the "package.json" file and add the start command so the "package.json" file will look like this:
{ "name": "myrestapi", "version": "1.0.0", "description": "my node api", "main": "app.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1", "start" : "node app.js" }, "author": "corey domingos", "license": "ISC", "dependencies": { "express": "^4.17.2" } }
Then create a file in the root directory "app.js", which will be executed when the start command defined in the "package.json" file is ran. In the "app.js" file we will implement the below code to start the sever listening on port "8080":
var express = require('express'); var app = express(); var port = 8080; app.listen(port, () => { console.log(`Server is listening on port: ${port}`) })
Execute the start command in your project folder:
node app.js
In your terminal you should see the below:
Server is listening on port: 8080
With just a couple steps, your first Node.js REST API is live on your localhost. Pretty easy right? Now lets set up one API endpoint to test. We will modify the existing "app.js" file with one "GET" endpoint like below:
var express = require('express'); var app = express(); var port = 8080; app.get('/testApi', (req, res) => { res.status(200).json({status: true, messsage : "The api is working!"}) }) app.listen(port, () => { console.log(`Server is listening on port: ${port}`) })
After restarting the server with the start command, navigate to your desired web browser and access "http://localhost:8080/testApi". If all went well you should see the below displayed on the webpage.
{"status":true,"messsage":"The api is working!"}
Now the simple REST API that we built in this demo does not require in depth testing and can be accessed in your browser — but as your API endpoints become more complex you'll need to make use of an API testing tool. The most common REST API tool is Postman, but there are plenty of other alternatives on the market to help you test your endpoints. My favorite REST API testing tool is Advanced Rest Client, which can be used as a desktop application, or chrome extension.
We now have a running REST API using Node.js and Express to build on. While we only created one endpoint in this post, going forward we will use this barebones project to develop a full CRUD (Create, Request, Update, Delete) REST API. Interested in creating a conversation on this blog post? Create a post in our Node.js community!
As long as we have been developing software applications, the oh-so-common phrase is xyz worth learning has been around. Well maybe not so much back in the C/Fortran days, but you get my gist. In 2022, this question is constantly asked as we have so many options when it comes to what to implement in your application. The hot topic for today, is learning Angular in 2022 worth your time? Or could you spend your time better learning another web framework?
Angular is web development framework that is built on TypeScript, and for those who are not familiar, TypeScript is JavaScript with the addition of types. This is good news for the developers that have existing skills with writing JavaScript, as picking up on the additional Types should be a breeze. For those who do not have at least foundational knowledge of JavaScript, learning TypeScript might prove to be more difficult. Angular is a component based web framework, that is typically beneficial to use when creating large web applications.
Since Angular is a component based web framework, these components in combination with one an other, will help us create a web application. For each component in an Angular project you will have a associated TypeScript class, in which you will be able to implement your component level logic. Aside from your TypeScript class you will have an associated HTML template to tell Angular how to render your component on the page, and if desired an optional CSS style sheet to define the look and feel of your component template.
Now I wouldn't go as far as to say that learning Angular takes an excessive amount of time and effort, but compared to other common web frameworks like React, the learning curve with Angular is going to be a little bit steeper. There are some deeper theories to Angular like Dependency Injection, which tend to confuse new comers, but after working with these nuances you'll be glad you chose to work with Angular for your next project. There are countless resources to assist in your journey learning Angular, and in my opinion they have some of the best docs which can be found here. And yes, just to clear up any confusion, these docs are actually useful!
Taking those first steps with a new technology is always stressful, but with Angular it is extremely easy to get your first app serving on your localhost. If you have node and npm already on your machine, half of the process is already done. If not, you can follow this link to download node, and in most cases npm will be downloaded along with node. Next we will need to download the Angular CLI using the following command:
npm install - g @angular/cli
Once the Angular CLI is downloaded, you will be able to create your first application by executing:
ng new myTestApp
Make sure to choose yes for routing, and to choose either CSS or SCSS for your style sheet. From this point you can open up your project directory and run either:
code .
or
ng serve
The "code ." command will open your current project in Visual Studio Code (my go to editor), and the "ng serve" command will start serving your first Angular application at localhost:4200.
And that's it! Your first project is created and serving all with 3 commands, excluding the command to open up your project in your editor. Pretty simple isn't it?
I may be biased when it comes to whether you should learn Angular, or to chose another alternative web framework, but my opinion is yes. Angular is my go to framework for both small and large web applications and the project structure/syntax is easy to work with once you become accustomed to it. By nature Angular is meant for large scale applications which makes scaling projects a breeze, which is always a thought in the back of my mind when starting a new project. With the addition of new packages like Angular Universal to assist with the many downfalls of SPA's not being compatible with modern SEO practices (if you're willing to give up CSR), and Ionic Angular for mobile application development, it's almost a no brainer to me. So if you're interested in creating highly scalable and easily readable modern web applications, I would definitely spend the time to learn Angular in 2022.
Interested in creating a discussion on this blog post? Start a conversation in our Web Development forum!
I found myself bouncing from platform to platform to stay up to date with the latest technologies and network with others in the IT Community, I kept thinking there must be a better way to do this. That's when the idea of creating a truly unrestricted centralized platform for the Software Development and IT Communities was born. So stick with me as we walk through the platform that the StackCafe Team created for you to use and enjoy.
- Ensure all community members have the ability to speak freely on our platform regardless of experience level.
- Make use of Moderation only when it is necessary.
- Never push our users to modify or delete any content that is not a community violation.
When you create an account with StackCafe you will be allowed access to post to your feed. Your feed will contain posts from other community members that you have followed and communities you have joined. Don't worry, you can mute posts showing in your feed for a given community if you wish. Your personalized feed will act as a great place for you to interact with other users, post your thoughts on a technical or non technical level, and stay up to date with any posts in your communities so you can lend a hand to others where you see fit.
Our platform offers 25+ relevant technical Question and Answer forums. In our communities there are no restrictions as to who can post, comment, and interact within our communities. We feel as though is was imperative to give all users, no matter their experience level a voice to ask questions, and provided answers where ever they see fit. StackCafe will never, and we mean never restrict your ability to interact in our community based off of your experience level or reputation score.
Our communities will allow any registered user, regardless if you are a member of that community the ability to post and interact with others posts. Users that join a given community will have the chance to rise to the top of the community leader board based off of your interactions within that community. Have a question, feel free to ask! If you receive a comment from another user that solved your problem, make sure to mark it as an answer to give them credit for their contribution!
Would StackCafe truly be a social media platform if we didn't allow our users to create a personalized profile? To some that may be true, but for the StackCafe team we felt it was necessary for us to allow our users to create a profile so other community members can have a good look into what makes you, you. Your profile will give you the ability to tell others basic information about yourself like your skills, education, and a profile image so you can put a face to your name. Completing your profile is not necessary to interact with other users, and is entirely optional.
Your profile will also display your community activity to other users. This means that any posts to your feed and posts in communities other users will be able to see, giving you credit for the content that you created on our platform. Additionally, other users will be able to see how many people you have impacted, your reputation score, and your stats within any communities that you are a member of. Don't want a particular user to be able to access your profile? On our platform we give every user the ability to restrict who can see their public profile.
We've all been there. You're doing your daily surf through your array of platforms that you use to stay up to date with the latest tech and you encounter a user that you want to interact with, only to find that you can't. On StackCafe, you'll never have that problem. Our built-in private messaging system allows you to connect with other users when you would like to allowing you to network and make connections with other like minded individuals.
So what are you waiting for? Create an account today!